Phoenix LiveView, hooks and push_event: json-view
Phoenix LiveView is a powerful framework built on Elixir, and offers an innovative approach to building real-time web interfaces without the need for extensive Javascript.
However, integrating with existing client-side libraries, like those for graphing, visualisation or rendering, can sometimes prove a challenge.
In this article, we’ll explore how we can integrate Phoenix LiveView with Javascript libraries that render to the DOM directly.
We’ll come across hooks, which allow us to run Javascript on certain lifecycle elements for a given element, and how these hooks enable streams of events (using push_event
in LiveView and pushEvent
in Javascript) to allow bi-directional real-time communication between client and server.
Background
TLDR: In Phoenix LiveView, you can use hooks and push_event to push data, and have the client-side library handle the rendering.
json-view (demo) - which displays JSON objects on a webpage as a tree that can be expanded and collapsed - is a good example of how some client-side Javascript libraries take control of rendering to the DOM and interaction with it.
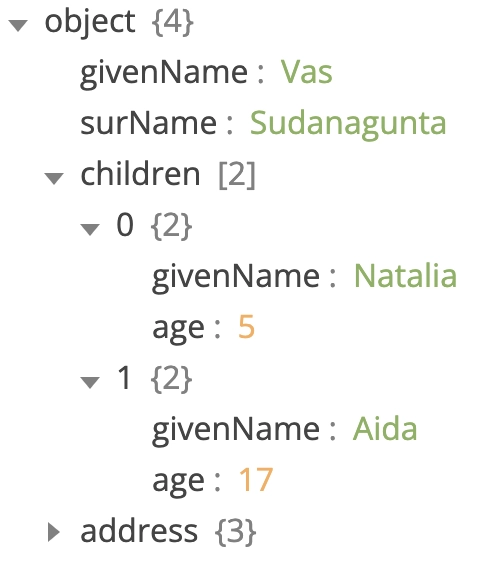
Let’s work through an example of how to integrate this with JSON data provided by the (LiveView) server. We’ll send some static data from the backend in response to an event, but this data could come from any source.
The following will assume you’ve set up a Phoenix project using mix phx.new, with LiveView enabled.
Library setup
There are multiple ways to incorporate a Javascript package as part of the page’s Javascript.
Recommending a preferred setup is outside the scope of this article, but there are two main ways:
assets/vendor
- in the typical LiveView project template,topbar
is placed in this directory and included in theassets/app/app.js
entrypoint file. This is suitable for smaller, more stable libraries in general, particularly if a single file version of the library is provided.NPM/Yarn -
esbuild
andwebpack
can pack referencednode_modules
file into a single distribution file. This is suitable for larger, more complex libraries that change more frequently.
The problem with json-view
There are two incompatible models of rendering when we try to combine the two libraries.
LiveView follows the declarative model - you design the view, determine what data should be displayed, and LiveView works out which elements of the page need to change when the underlying data is changed.
It achieves this by using socket assigns in render
:
1def render(assigns) do2 ~H"""3 <p>Hello <%= @name %></p>4 """5 end
However, libraries like json-view
work on the imperative model. We specify the steps required to display the data in Javascript, outside the layout of the DOM itself:
1import jsonview from '@pgrabovets/json-view';2 3const data = '{"name": "json-view", "version": "1.0.0"}'4const tree = jsonview.create(data);5jsonview.render(tree, document.querySelector('.tree'));
These two models are at odds. We have seemingly no way to match up the two methods of rendering data (declarative and imperative), but we need libraries like json-view
to render rich interfaces on the client.
Fundamentally - we need to run Javascript code when the server prompts a change of page state. Let’s take a look at hooks, which helps to reconcile these two rendering models.
Hook setup
In LiveView, hooks are the way to provide bidirectional communication between the (browser) client and (LiveView) server, with the core object of communication being the event.
Hooks are defined in the LiveSocket
and attached to an element using the phx-hook
attribute. There are a number of callbacks - but we’ll focus on the mounted
callback, since we can co-ordinate everything else via events. You can supply callbacks for other life-cycle events too - check the docs.
Within a callback like mounted
, the hook sends events to the backend using this.pushEvent
, and handles events from the server by registering a handler for a particular event name using this.handleEvent
.
It’s important to note only one hook is permitted per element, so you only need to reason about one stream of events between client and server.
With that knowledge in mind - let’s define a JsonView
hook that registers an event handler, that eventually calls jsonview.render
on the element to render the tree of data.
1import { Socket } from 'phoenix'; 2import jsonview from '@pgrabovets/json-view'; 3 4const JsonViewHook = { 5 mounted() { 6 this.handleEvent("render_json", ({ data }) => { 7 this.el.innerHTML = ""; 8 const tree = jsonview.create(data); 9 jsonview.render(tree, this.el);10 });11 }12}13 14const liveSocket = new LiveSocket(15 "/live",16 Socket,17 {hooks: { JsonView: JsonViewHook }, ...}18 );19...
We do several things in these several lines of code:
We define a
JsonViewHook
object with amounted
function. This function will be called when the element with this hook is mounted in the DOM.Inside
mounted
, we set up an event listener for a custom event called "render_json". This event will be triggered from our LiveView.When the event is received, it expects a
data
parameter containing the JSON to be rendered.We clear the existing content of the element.
We use
jsonview.create
andjsonview.render
to render the JSON data into our element.
To use this hook - we’ll just need to add the phx-hook
attribute with the name of the hook (”JsonView”
) to an element:
1<div id="json-container" phx-hook="JsonView"></div>
Sending events to the server
We’ll just need to trigger an event from the backend to provide this data. We’ll leave this outside the scope of this article for now - perhaps a button could trigger an event to the backend? - but you could use this.pushEvent
from the mounted
hook like so:
1mounted() {2 this.pushEvent("send_json", {});3}
to send an event to the LiveView server that can be handled with handle_info
. The relevant section of the LiveView documentation covers more possibilities with this.pushEvent
, including a scenario where a handler function can be specified to handle a reply payload directly.
Sending events from the server
push_event/3 is the way to push an event from LiveView to the browser. It can be called at any point - including in mount
- though a good practice is to ensure that the page and all its elements are in a known state before sending these events. Otherwise - you’ll have events silently being dropped during page setup - a sure route to unpredictability!
Let’s write a handle_event
clause for an event we might receive from the client, which pushes an event to the client:
1def handle_event("send_json", _params, socket) do2 {:noreply, push_event(socket, "render_json", %{data: [1, 2, 3]})}3end
That’s it! And there’s flexibility here too. Registering event handlers with names on both the client and the server marks a clear separation between the handling of the event and how the event is raised.
Further integration
We’ve seen a simple example of the integration between client and server. A common extension of this would be to scope certain updates to certain elements, through the use of event parameters and element attributes. This helps to reduce the amount of unnecessary events and handler work.
This event handling can also be expanded for tighter client-side library integration with the backend. For example, typically these Javascript libraries emit higher-level user interaction events. Our library example, json-view
, doesn’t do this, but a charting library like chart.js does.
However, from a user interaction standpoint, a round-trip to the server for processing should be generally avoided. Typically, any rendering update based on events would be handled by the rendering library client-side.
But capturing user activity for other reasons is a common use case. This includes monitoring, logging and analysis. These don’t require a response, so pushEvent
from within a hook can be ideal for this type of asynchronous processing on the server.
Conclusion
Integrating powerful Javascript client-side libraries that require control over the DOM is a key part of creating rich, dynamic user interfaces. Not all page updates need to be real-time, and so retaining LiveView offers a powerful yet simple way to continue to control other page state.
Becoming familiar with LiveView hooks and their associated events makes integrating these with data that originates from the server possible. It’s also important to note that not all user interactivity require a round-trip to the server, and the interfaces you build will be more flexible and responsive when you use powerful Javascript libraries.